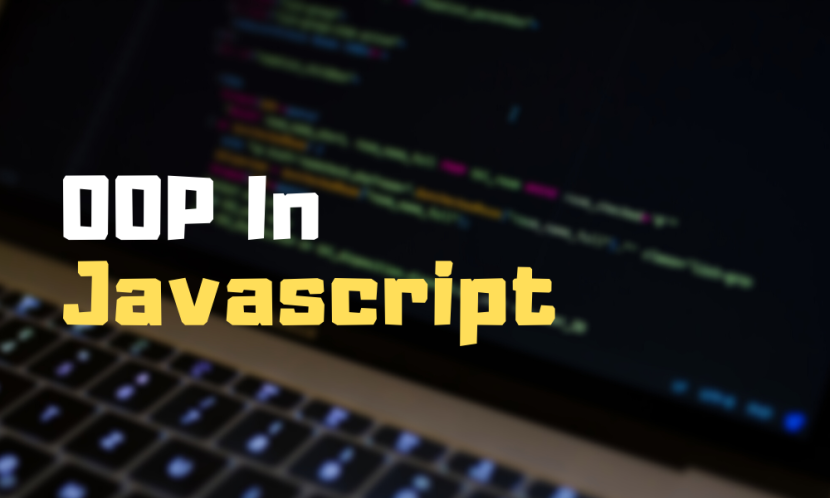
Mastering Object-Oriented Programming (OOP) in JavaScript: Concepts, Best Practices, and Applications
Introduction
Object-oriented programming (OOP) is a programming paradigm that uses objects and classes to structure software in a way that models real-world entities. This approach enhances code reusability, scalability, and maintainability. JavaScript, a versatile language for both frontend and backend development, supports OOP principles, making it essential for developers to grasp these concepts.
In this comprehensive guide, we'll delve into the fundamentals of OOP in JavaScript, covering core concepts, best practices, and practical examples.
Key Concepts of OOP in JavaScript
1. Classes and Objects
Classes are blueprints for creating objects. They encapsulate data (properties) and functions (methods) that operate on the data. Objects are instances of classes.
Example:
class Car {
constructor(brand, model) {
this.brand = brand;
this.model = model;
}
displayInfo() {
return `${this.brand}${this.model}`;
}
}
const myCar = newCar("Toyota", "Corolla");
console.log(myCar.displayInfo()); // Output: Toyota Corolla
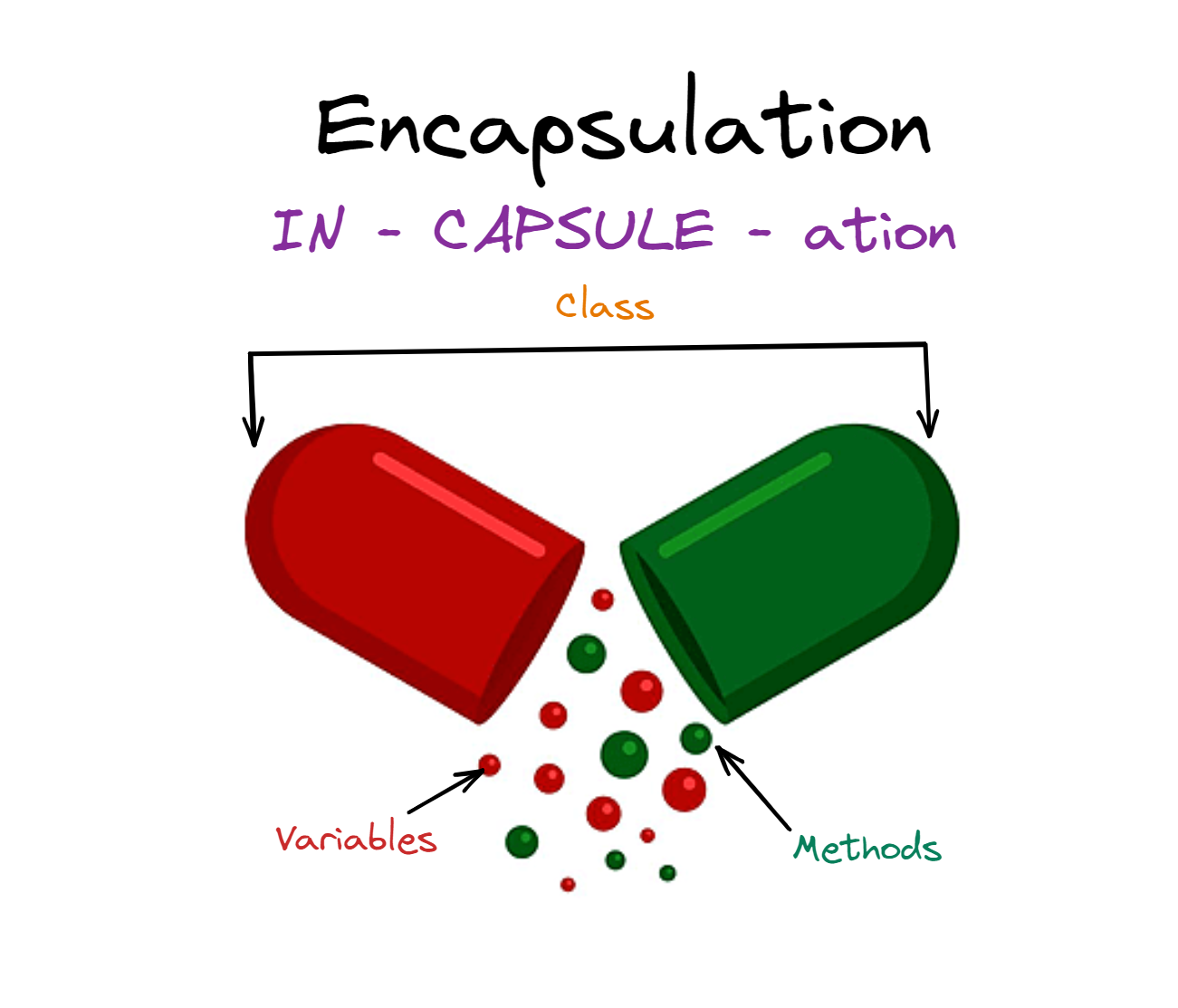
2. Encapsulation
Encapsulation involves bundling the data and methods that operate on the data within one unit, and restricting access to some of the object's components. This is achieved using private properties and methods.
Example:
classBankAccount {
#balance;
constructor(initialBalance){
this.#balance = initialBalance;
}
deposit(amount){
this.#balance += amount;
}
getBalance() {
return this.#balance;
}
}
const account = newBankAccount(1000);
account.deposit(500);
console.log(account.getBalance(
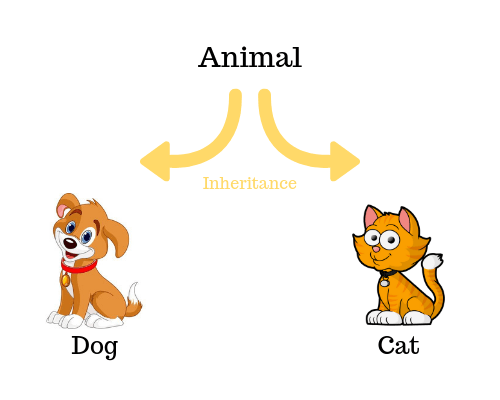
3. Inheritance
Inheritance allows a class to inherit properties and methods from another class. This promotes code reusability.
Example:
classAnimal
{
constructor(name){
this.name = name;
}
makeSound(){
return`${this.name} makes a sound.`;
}
}
classDogextendsAnimal {
makeSound(){
return`${this.name} barks.`;
}
}
const myDog = newDog('Buddy'); console.log(myDog.makeSound()); // Output: Buddy barks.
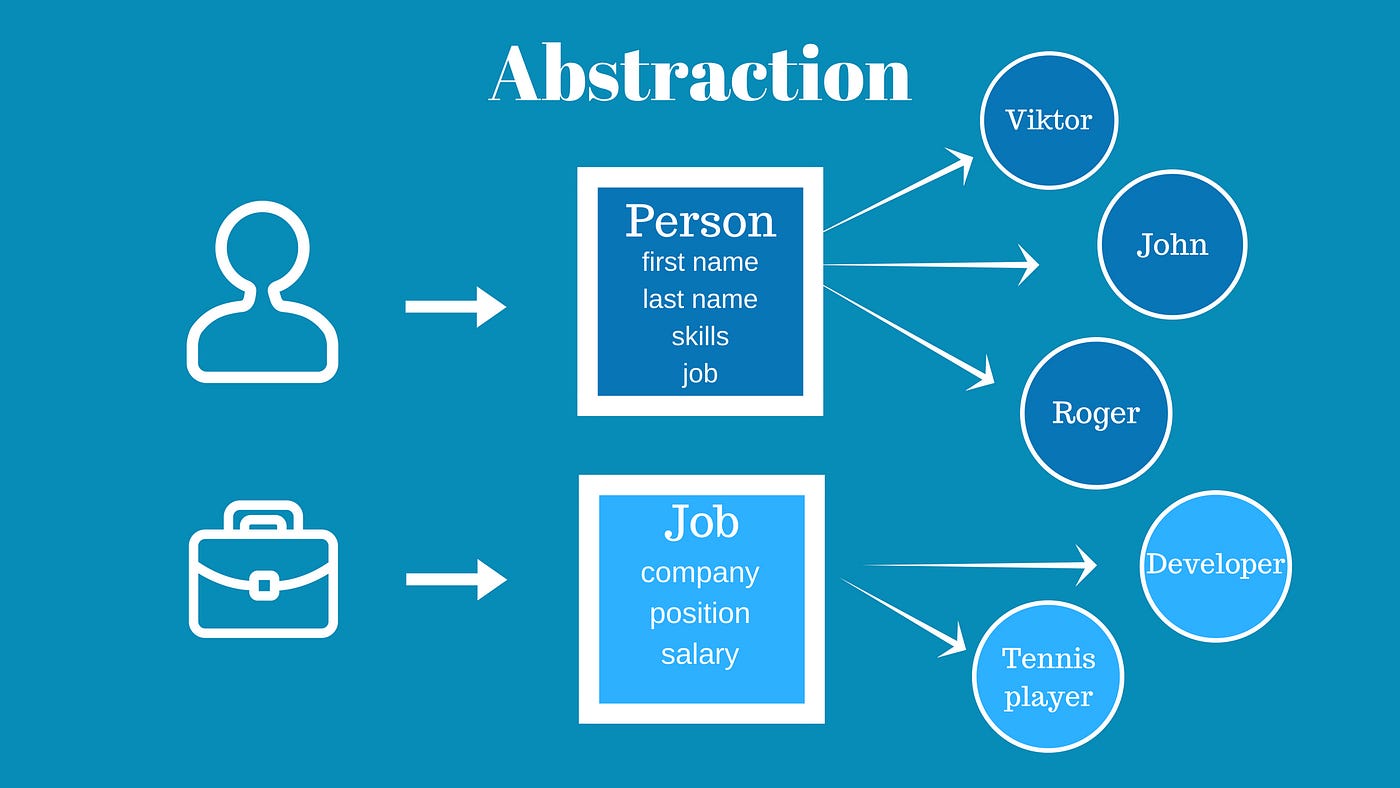
4. Abstraction
Abstraction simplifies complex systems by modeling classes appropriate to the problem, while hiding irrelevant details. This is usually implemented using abstract classes or interfaces.
Example:
classVehicle {
constructor(type){
this.type = type;
}
startEngine(){
thrownewError('You must implement startEngine method');
}
}
classCarextendsVehicle {
startEngine(){
return`${this.type} engine started.`;
}
}
const myCar = newCar('Sedan'); console.log(myCar.startEngine()); // Output: Sedan engine started.
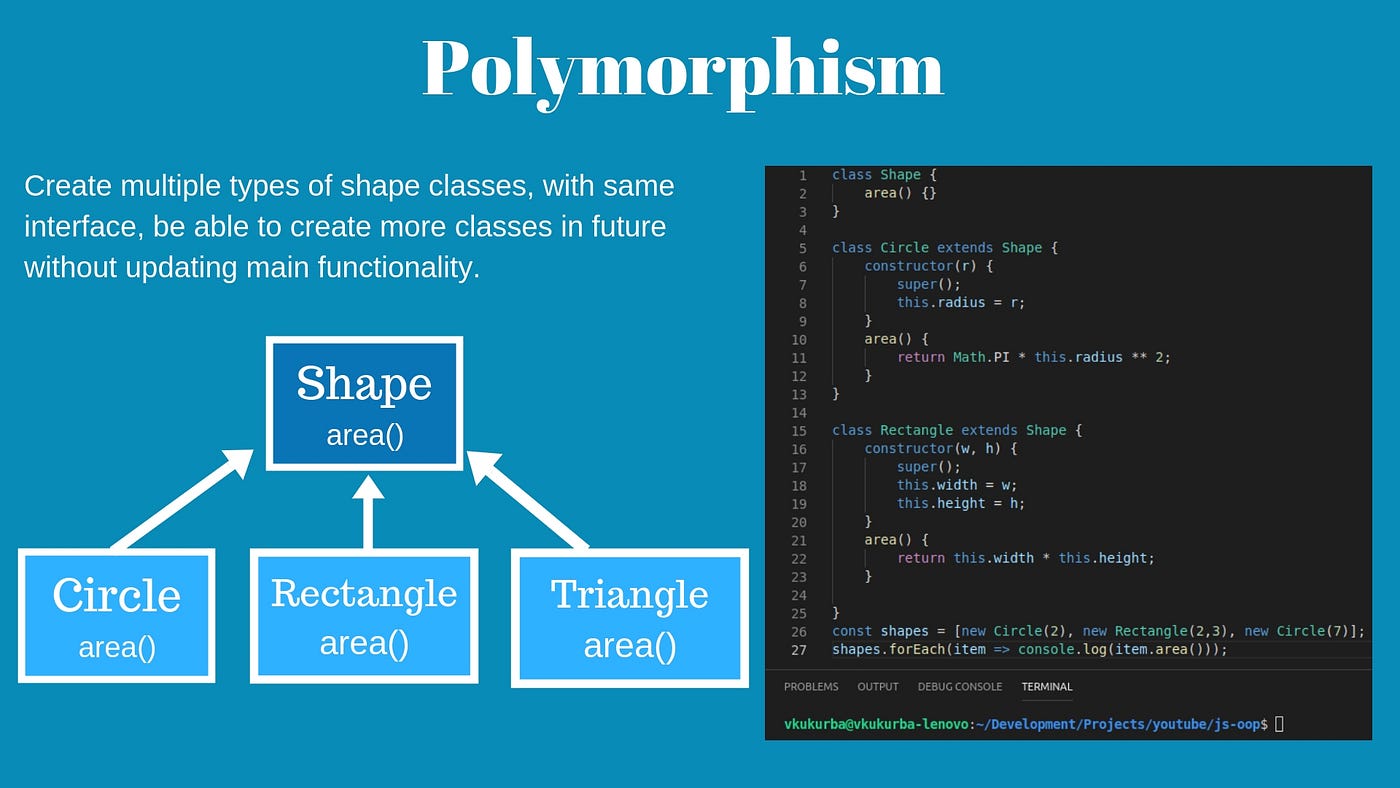
5. Polymorphism
Polymorphism allows methods to do different things based on the object it is acting upon. It is often achieved through method overriding.
Example:
classBird {
fly(){
return'Bird is flying';
}
}
classPenguinextendsBird {
fly(){
return'Penguins cannot fly';
}
}
const myBird = newBird();
const myPenguin = newPenguin(); console.log(myBird.fly()); // Output: Bird is flyingconsole.log(myPenguin.fly()); // Output: Penguins cannot fly
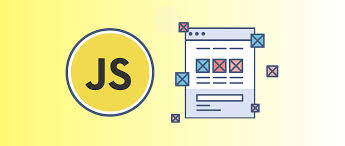
Best Practices for OOP in JavaScript
- Keep Classes Focused and Simple: Each class should have a single responsibility and be as simple as possible.
- Use Encapsulation Wisely: Protect the internal state of your objects and only expose what is necessary.
- Favor Composition Over Inheritance: Use composition to combine objects rather than relying solely on inheritance.
- Leverage ES6 Features: Use modern JavaScript features like classes, modules, and arrow functions to write cleaner and more efficient code.
- Adopt SOLID Principles: Follow the SOLID principles (Single Responsibility, Open/Closed, Liskov Substitution, Interface Segregation, Dependency Inversion) to create robust and maintainable code.
Practical Applications of OOP in JavaScript
- Building Web Applications: Use OOP principles to create modular and reusable components in frameworks like React, Angular, or Vue.
- Server-Side Development: Apply OOP concepts in backend frameworks like Node.js and NestJS to create scalable server-side applications.
- Game Development: Utilize OOP to manage game objects, interactions, and game state in JavaScript-based game engines.
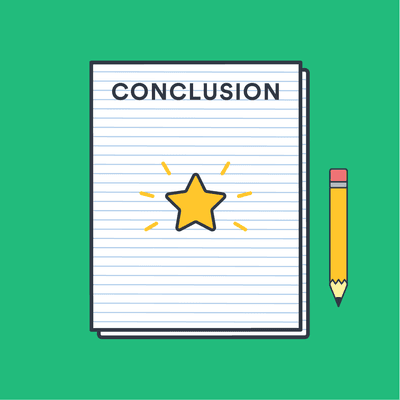
Conclusion
Mastering Object-Oriented Programming in JavaScript is crucial for developing robust, maintainable, and scalable applications. By understanding and applying core OOP concepts such as classes, encapsulation, inheritance, abstraction, and polymorphism, you can significantly enhance your coding skills and build more efficient software solutions. Remember to follow best practices and leverage modern JavaScript features to write clean and effective code.
Further Reading and Resources
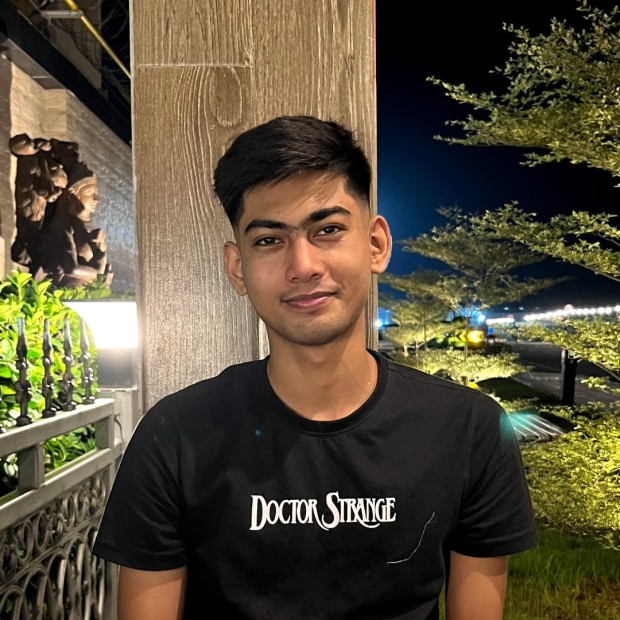
Article by s1lent
Published 02 Aug 2024