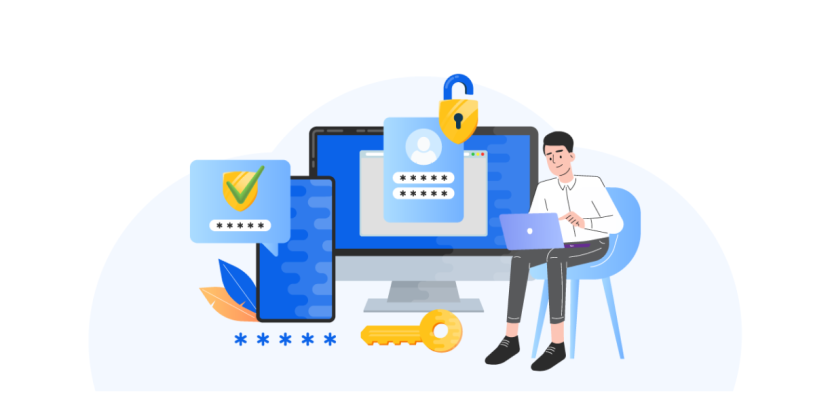
Mastering Authentication in Node.js and Express: Best Practices, Tools, and Common Pitfalls
Introduction
Authentication is the backbone of web security, ensuring that only authorized users gain access to sensitive information and functionalities. In the fast-evolving landscape of web development, mastering authentication in Node.js and Express is crucial for developers aiming to build secure and scalable applications.
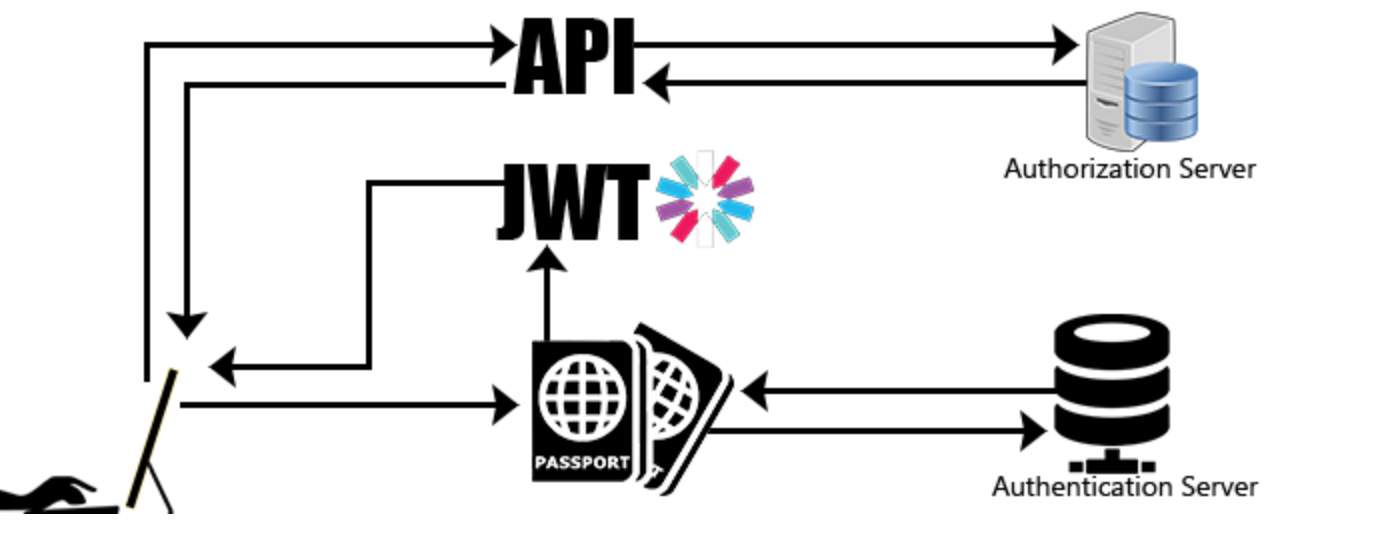
Essential Authentication Libraries for Node.js and Express
To implement robust authentication, several libraries stand out:
Passport.js
- Overview: A popular and flexible authentication middleware for Node.js.
- Features: Supports over 500 authentication strategies, including local, OAuth, and OpenID.
- Why Use It?: Its modular approach allows easy integration with different authentication mechanisms.
JSON Web Token (JWT)
- Overview: A compact, URL-safe means of representing claims to be transferred between two parties.
- Features: Self-contained tokens with embedded user information.
- Why Use It?: Ideal for stateless authentication in RESTful APIs.
bcrypt
- Overview: A library to help you hash passwords.
- Features: Incorporates salting to protect against rainbow table attacks
- Why Use It?: Ensures stored passwords are secure and hard to crack
Best Practices for Secure Authentication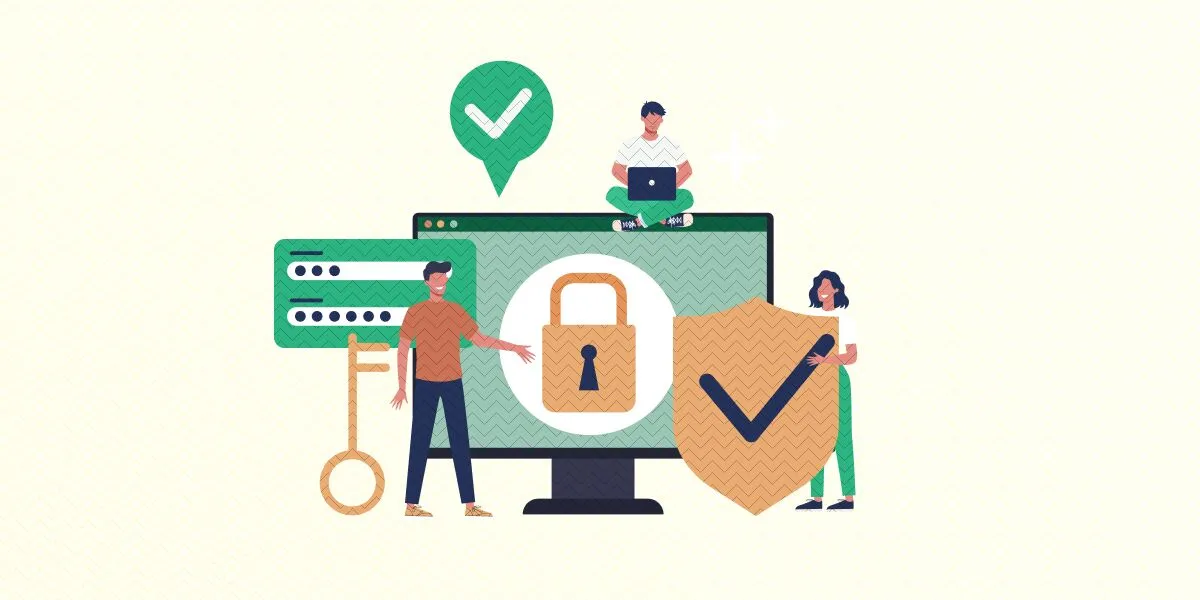
Implementing authentication goes beyond just using libraries; it requires following best practices to ensure your application remains secure.
Storing Passwords Securely
- Use Strong Hashing Algorithms: Always hash passwords using algorithms like
bcrypt
- Salting Passwords: Enhance security by adding a unique salt to each password before hashing.
Managing Secrets and Environment Variables
- Environment Variables: Store sensitive information such as secret keys in environment variables, not in your source code
- Configuration Management: Use configuration management tools to handle different environments (development, testing, production)
Session Management
- Secure Cookies: Use secure and HTTP-only cookies to store session information.
- Session Expiry: Implement appropriate session expiration to minimize the risk of session hijacking.
Protecting Routes
- Role-Based Access Control (RBAC): Implement RBAC to ensure users only access resources they are authorized to
- Middleware for Authentication: Use middleware to protect routes and ensure that only authenticated users can access certain endpoints.
Error Handling
- Meaningful Error Messages: Provide clear and secure error messages that do not expose sensitive information
- Consistent Error Handling: Implement a consistent error-handling strategy across your application.
Common Pitfalls to Avoid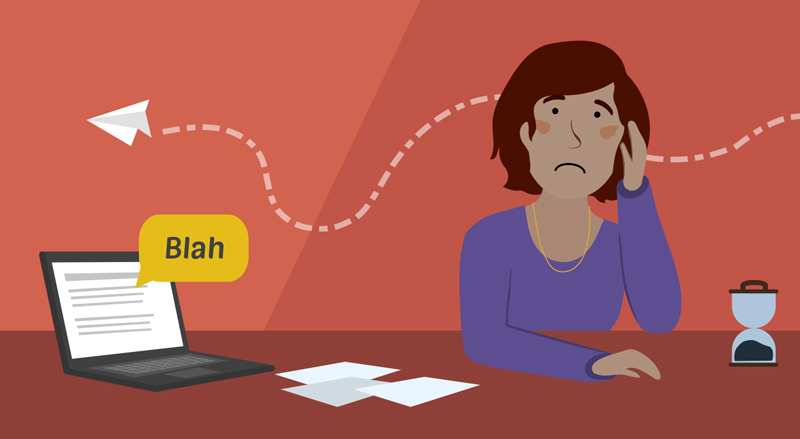
While implementing authentication, developers often encounter common pitfalls that can compromise security and user experience.
Storing Plaintext Passwords
Storing passwords in plaintext is a significant security risk. Always hash and salt passwords before storing them in your database
Hardcoding Secrets
Embedding secrets like API keys and passwords in your source code can lead to security breaches if your codebase is exposed. Use environment variables instead.
Inadequate Session Management
Not setting secure attributes for cookies or having long session expiry times can make your application vulnerable to attacks.
Overlooking Error Handling
Improper error handling can leak sensitive information or confuse users. Ensure your error messages are user-friendly but do not reveal technical details.
Conclusion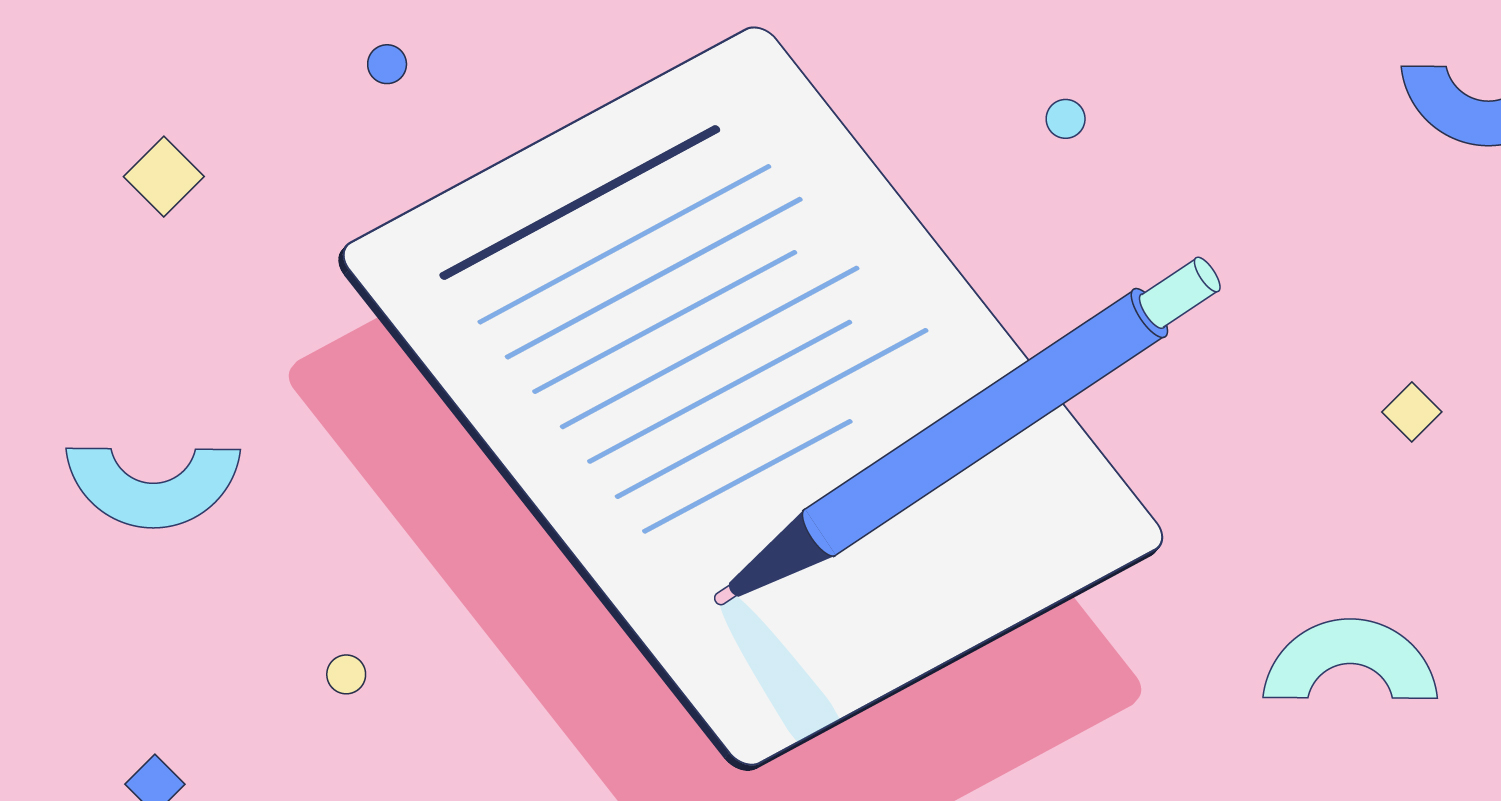
Mastering authentication in Node.js and Express is essential for building secure and robust web applications. By leveraging the right tools and adhering to best practices, you can protect your application from common security threats and provide a seamless user experience. Stay vigilant and continuously update your knowledge to keep up with the ever-evolving security landscape.
Further Reading and Resources
Passport.js Documentation
JSON Web Token (JWT) Introduction
Express.js Security Best Practices
Videos
Watch the full extensive guide here -
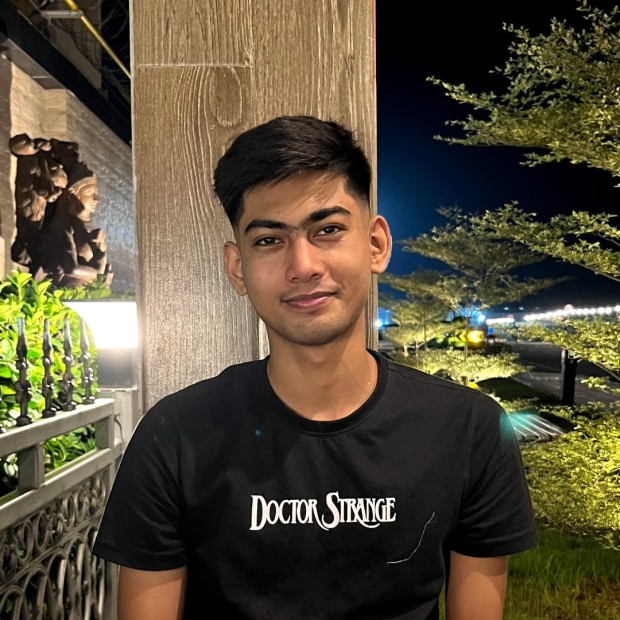
Article by s1lent
Published 08 Jul 2024