100 Javascript interview Questions with answers
- What is closure in JavaScript?
- What is the difference between null and undefined in JavaScript?
- Can you explain the difference between == and === in JavaScript?
- Can you explain hoisting in JavaScript?
- Can you explain how to implement inheritance in JavaScript?
- What is the difference between let and const in JavaScript?
- Can you explain how to implement a Promise in JavaScript?
- Can you explain how to handle errors in JavaScript?
- Can you explain the event bubbling and capturing mechanism in JavaScript?
- Can you explain the difference between synchronous and asynchronous code in JavaScript?
- Can you explain the concept of prototypes in JavaScript?
- Can you explain the concept of a callback function in JavaScript?
- Can you explain how to implement the Singleton pattern in JavaScript?
- Can you explain how to implement a Factory pattern in JavaScript?
- Can you explain how to implement the Observer pattern in JavaScript?
- Can you explain the difference between a forEach loop and a for loop in JavaScript?
- Can you explain how to implement a Map in JavaScript?
- Can you explain how to implement a Set in JavaScript?
- Can you explain how to implement a WeakMap in JavaScript?
- Can you explain how to implement a WeakSet in JavaScript?
- Can you explain the concept of the this keyword in JavaScript?
- Can you explain how to implement a decorator in JavaScript?
- Can you explain how to implement a class in JavaScript?
- Can you explain how to implement a module in JavaScript?
- Can you explain how to implement a mixin in JavaScript?
- Can you explain how to implement a generator function in JavaScript?
- Can you explain how to implement an async/await function in JavaScript?
- Can you explain how to implement a recursive function in JavaScript?
- Can you explain how to implement a memoization in JavaScript?
- Can you explain how to implement a tail call optimization in JavaScript?
- Can you explain how to implement a destructuring assignment in JavaScript?
- Can you explain how to implement a spread operator in JavaScript?
- Can you explain how to implement a rest parameter in JavaScript?
- Can you explain how to implement a default parameter in JavaScript?
- Can you explain how to implement a template literal in JavaScript?
- Can you explain how to implement a tagged template literal in JavaScript?
- Can you explain how to implement a typeof operator in JavaScript?
- Can you explain how to implement an instanceof operator in JavaScript?
- Can you explain how to implement a new operator in JavaScript?
- Can you explain how to implement an Object.create method in JavaScript?
- Can you explain how to implement an Object.assign method in JavaScript?
- Can you explain how to implement an Object.entries method in JavaScript?
- Can you explain how to implement an Object.values method in JavaScript?
- Can you explain how to implement an Object.keys method in JavaScript?
- Can you explain how to implement an Array.map method in JavaScript?
- Can you explain how to implement an Array.filter method in JavaScript?
- Can you explain how to implement an Array.reduce method in JavaScript?
- Can you explain how to implement an Array.sort method in JavaScript?
- Can you explain how to implement an Array.reverse method in JavaScript?
- Can you explain how to implement an Array.concat method in JavaScript?
- Can you explain how to implement an Array.slice method in JavaScript?
- Can you explain how to implement an Array.splice method in JavaScript?
- Can you explain how to implement an Array.push method in JavaScript?
- Can you explain how to implement an Array.pop method in JavaScript?
- Can you explain how to implement an Array.shift method in JavaScript?
- Can you explain how to implement an Array.unshift method in JavaScript?
- Can you explain how to implement an Array.indexOf method in JavaScript?
- Can you explain how to implement an Array.includes method in JavaScript?
- Can you explain how to implement an Array.find method in JavaScript?
- Can you explain how to implement an Array.findIndex method in JavaScript?
- Can you explain how to implement a Math.max method in JavaScript?
- Can you explain how to implement a Math.min method in JavaScript?
- Can you explain how to implement a Math.random method in JavaScript?
- Can you explain how to implement a Math.floor method in JavaScript?
- Can you explain how to implement a Math.ceil method in JavaScript?
- Can you explain how to implement a Math.round method in JavaScript?
- Can you explain how to implement a parseInt function in JavaScript?
- Can you explain how to implement a parseFloat function in JavaScript?
- Can you explain how to implement a isNaN function in JavaScript?
- Can you explain how to implement a isFinite function in JavaScript?
- Can you explain how to implement a Number method in JavaScript?
- Can you explain how to implement a String method in JavaScript?
- Can you explain how to implement a Boolean method in JavaScript?
- Can you explain how to implement a Symbol method in JavaScript?
- Can you explain how to implement a RegExp method in JavaScript?
- Can you explain how to implement a Date method in JavaScript?
- Can you explain how to implement a JSON.stringify method in JavaScript?
- Can you explain how to implement a JSON.parse method in JavaScript?
- Can you explain how to implement a setTimeout method in JavaScript?
- Can you explain how to implement a setInterval method in JavaScript?
- Can you explain how to implement a clearTimeout method in JavaScript?
- Can you explain how to implement a clearInterval method in JavaScript?
- Can you explain how to implement a function expression in JavaScript?
- Can you explain how to implement a function declaration in JavaScript?
- Can you explain how to implement a function constructor in JavaScript?
- Can you explain how to implement a function bind method in JavaScript?
- Can you explain how to implement a function call method in JavaScript?
- Can you explain how to implement a function apply method in JavaScript?
- Can you explain how to implement a named function in JavaScript?
- Can you explain how to implement an anonymous function in JavaScript?
- Can you explain how to implement a self-invoking function in JavaScript?
- Can you explain how to implement a function closure in JavaScript?
- Can you explain how to implement a function memoization in JavaScript?
- Can you explain how to implement a function currying in JavaScript?
- Can you explain how to implement a function composition in JavaScript?
- Can you explain how to implement a function pipe in JavaScript?
- Can you explain how to implement a function chaining in JavaScript?
- Can you explain how to implement a function partial application in JavaScript?
- Can you explain how to implement a function higher-order function in JavaScript?
- Can you explain how to implement a function decorator in JavaScript?
ANSWERS
What is closure in JavaScript?
A closure is a function that has access to variables in its outer scope, even after the outer function has returned. This allows for the preservation of state and the ability to create private variables within a function.
Real life use case: A closure can be used to create a private counter in a function, which can only be modified and accessed by that function.
function counter(){let count = 0; return function(){count++; return count; }}const myCounter = counter(); console.log(myCounter()); // 1 console.log(myCounter()); // 2 console.log(myCounter()); // 3
What is the difference between null and undefined in JavaScript?
null
is a value that represents the intentional absence of any object value. It is often used to indicate that an object reference has been intentionally cleared. undefined
is a value that indicates that a variable has been declared but has not yet been assigned a value.
Real life use case: A variable can be declared as null
to indicate that it will be used later to reference an object, while a variable that has not been assigned a value will be undefined
.
let obj = null; let name; console.log(obj); // null console.log(name); // undefined
Can you explain the difference between == and === in JavaScript?
==
performs type coercion, meaning that it will attempt to convert the operands to the same type before performing the comparison. ===
performs strict equality, meaning that it will only return true
if the operands are of the same type and have the same value.
Real life use case: In most cases, it is recommended to use ===
instead of ==
for reliable and predictable comparison results.
console.log(1 =='1'); // true console.log(1 ==='1'); // false
Can you explain hoisting in JavaScript?
Hoisting is a behavior in JavaScript where variables and function declarations are moved to the top of their respective scopes, before code execution. This means that variables and functions can be used before they are declared in the code.
Real life use case: Consider the following code, where the variable x
is declared after it is used in the code:
console.log(x); // undefined var x = 5;
Due to hoisting, the JavaScript engine will interpret the code as follows:
var x; console.log(x); // undefined x = 5;
Can you explain how to implement inheritance in JavaScript?
Inheritance in JavaScript can be implemented using prototypes. A prototype is an object that is used as a blueprint for creating new objects. When a new object is created, it can inherit properties and methods from its prototype.
Real life use case: Consider the following code, where a Person
prototype is used to create a new Developer
object that inherits properties and methods from the Person
prototype:
function Person(name, age){this.name = name; this.age = age; }Person.prototype.getInfo = function(){return `Name: ${this.name}, Age: ${this.age}`; }function Developer(name, age, skills){Person.call(this, name, age); this.skills = skills; }Developer.prototype = Object.create(Person.prototype); Developer.prototype.constructor = Developer; Developer.prototype.getSkills = function(){return this.skills; }const john = new Developer('John Doe', 30, ['JavaScript', 'Node.js', 'React']); console.log(john.getInfo()); // Name: John Doe, Age: 30 console.log(john.getSkills()); // [ 'JavaScript', 'Node.js', 'React' ]
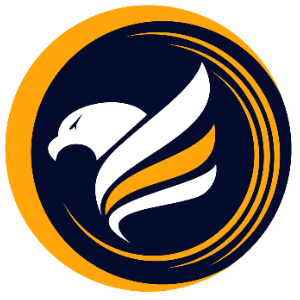
Article by Masynctech Coding School
Published 12 Feb 2023